Hi!
I write code on java for sending push notifications to users who did not go to the game more than a day. I worked in Eclipse Mars, OSX 10.11.2. Here is code (without libs):
public class Main {
/**
* Main Method Send Push Notification from Cloud
* @param args
*/
public static final void main(String[] args) {
getRecipients();
}
static final void getRecipients() {
long currentTimeInMinutes = Clock.systemUTC().millis()/60000;
String dbName = "...";
String collectionName = "...";
App42API.initialize("...","...");
StorageService storageService = App42API.buildStorageService();
Storage storage = storageService.findAllDocuments(dbName,collectionName);
ArrayList<Storage.JSONDocument> jsonDocList = storage.getJsonDocList();
for(int i=0;i<jsonDocList.size();i++)
{
String jsonString = jsonDocList.get(i).getJsonDoc();
String docID = jsonDocList.get(i).getDocId();
JSONObject jobj = null;
try {
jobj = new JSONObject(jsonString);
} catch (JSONException e) {
e.printStackTrace();
}
long lastLoadedTime = 0;
try {
lastLoadedTime = jobj.getLong("lastLoadedTime");
} catch (JSONException e) {
e.printStackTrace();
}
if(currentTimeInMinutes - lastLoadedTime > 1440)
try {
sendPN(jobj.getString("userName"));
jobj.put("lastLoadedTime",currentTimeInMinutes);
storage = storageService.addOrUpdateKeys(dbName,collectionName,docID,jobj);
} catch (JSONException e) {
e.printStackTrace();
}
}
}
static final void sendPN(String userName) {
String message = "Get free BribeMe, extra points or double time for bonuses!";
PushNotificationService pushNotificationService = App42API.buildPushNotificationService();
pushNotificationService.sendPushMessageToUser(userName, message);
}
};
I checked with the local computer everything works well. But I can't upload this to Custom code. If I make .jar using Eclipse, I get the message "Package can not be used in custom code deployment Main : in source Main". If I make .jar using
jar cf App42ServerPN.jar *
I get the message "Internal Server Error". What I'm doing wrong?
P.S. Also I can't increment the badge using java code. Becouse "Send Custom Push Message To User In Key Value Or JSON Format" option is unavailable in java. Is there any way to push notification from Custom code and increase badge counter on the iOS devices?
Thank you in advance.
P.P.S. I get Error in both cases. Using AppHQ I get this:
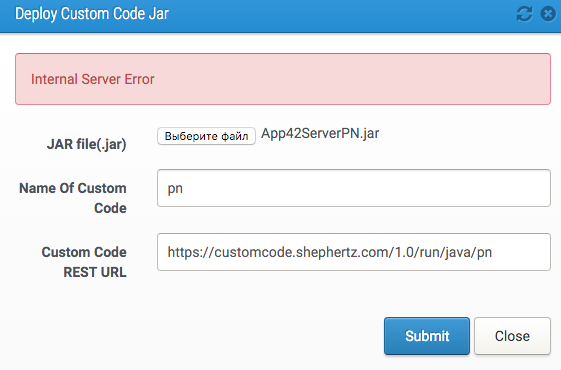
When I run the deploy code from Eclipse, I get this message:
Exception in thread "main" com.shephertz.app42.paas.sdk.java.App42Exception: {"app42Fault":{"httpErrorCode":500,"appErrorCode":1500,"message":"Internal Server Error","details":"Internal Server Error. Please try again"}}
at com.shephertz.app42.paas.sdk.java.util.Util.multiPartRequest(Util.java:358)
at com.shephertz.app42.paas.sdk.java.customcode.CustomCodeService.deployJarFile(CustomCodeService.java:82)
at Main.main(Main.java:21)
Thank you for response!